Introduction
cURL, short for Client URL, is a nifty command-line tool that simplifies transferring data with URLs. Whether fetching a web page, downloading files, or interacting with APIs, cURL covers you. It’s like your trusted sidekick in the world of web development, always ready to execute your commands and data mining from the internet.
Now, let’s talk about HTTP requests and headers. When your browser sends a request to a web server, it’s not just asking for a webpage; it’s also sending along some extra information called headers. This is what forms the basis for the cURL send header. These headers act like little notes attached to your request, telling the server what content you expect, who you are, and how you want to be authenticated.
So, if you’ve ever wondered how to send custom headers with cURL, you’re in the right place. By the end of this guide, you’ll be sending HTTP requests with headers like a seasoned developer. This will allow you to unlock new possibilities and take your web development game to the next level.Â
So, let’s dive in and master the art of sending HTTP headers with cURL!
What to Know About HTTP Headers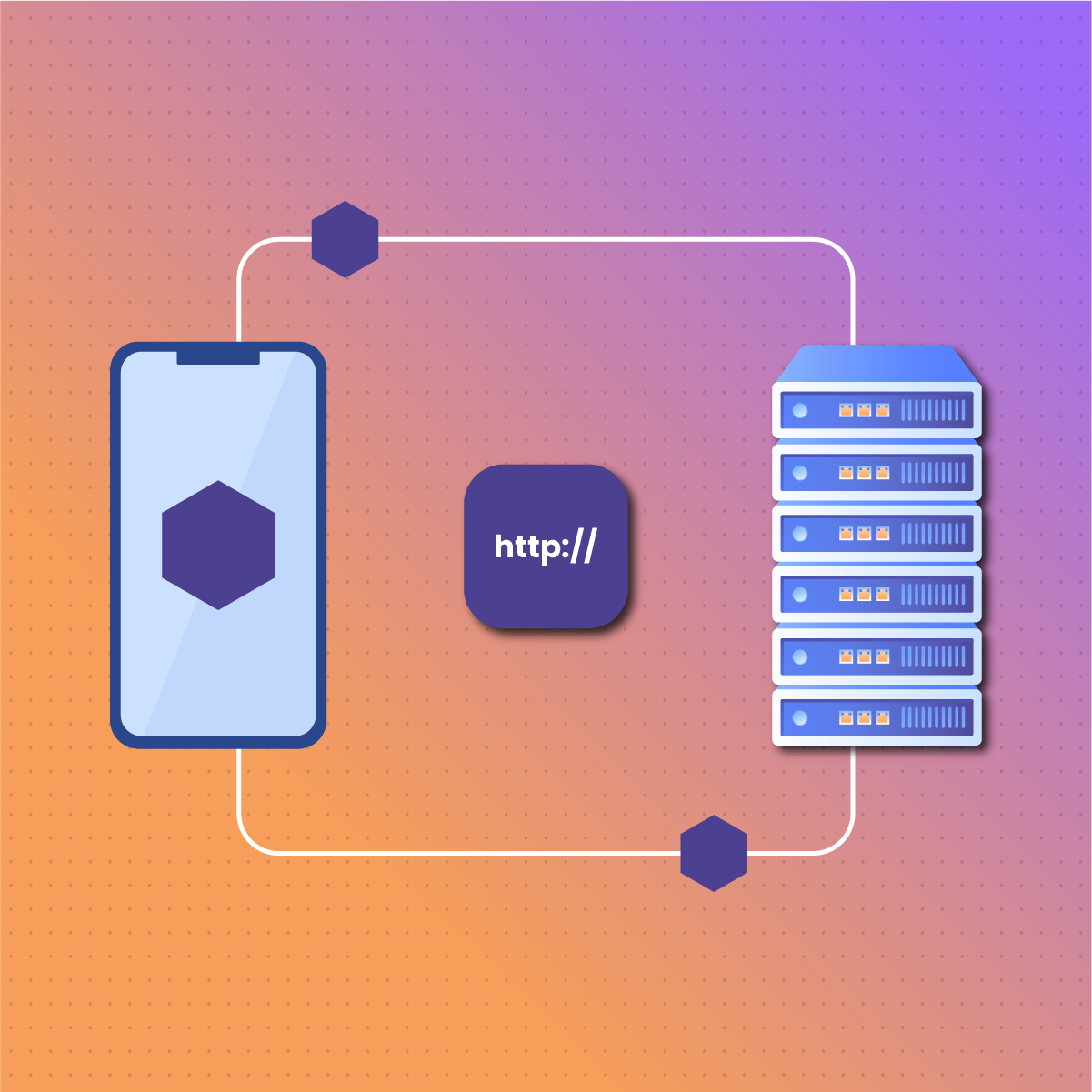
HTTP headers are messages passed between your browser and a web server during a conversation. They carry important information about the request or response, helping both sides understand each other better. Think of them as the secret notes exchanged between friends, filled with valuable insights and instructions.
When you visit a website or make an API call, your browser sends an HTTP request to the server. Along with the request, it attaches headers containing details such as the type of content it’s looking for, the browser it’s using, and any cookies it wants to share. On the other hand, when the server responds, it also includes headers to indicate things like the content type, caching instructions, and whether the request was successful.
Explanation of Key-Value Pairs and Common HTTP Headers
HTTP headers are structured as key-value pairs, each consisting of a name and a value separated by a colon. For example, the Content-Type header might have a value of application/JSON, indicating that the content being sent or received is in JSON format.
Some common HTTP headers you’ll come across include:
- User-Agent: This identifies the browser or client making the request.
- Content-Type: It specifies the type of content being sent or received (e.g., text/html, application/JSON).
- Authorization: This provides credentials for authentication purposes.
- Accept: Indicates the type of content the client is willing to accept from the server.
- Cookie: Cookies store information about the client’s session.
These are just a few examples, but many more headers exist. Each serves its unique purpose in the grand scheme of web communication.
Significance of HTTP Headers for Various Functionalities
HTTP headers play a crucial role in enabling various functionalities in web communication. Here are some key functionalities and how headers contribute to them:
Authentication
Headers like Authorization allow clients to send credentials (such as API tokens or login information) to servers for authentication. This helps ensure that only authorized users can access protected resources.
Content Negotiation
The Accept header allows clients to specify the type of content they prefer to receive from the server. This helps servers deliver content in the most suitable format for the client, enhancing the user experience.
Caching Directives
Headers like Cache-Control and Expires instruct clients and servers on how to cache responses. By controlling caching behavior, headers help improve performance and reduce server load.
In summary, understanding the significance of HTTP headers is essential for effective web development. This understanding provides the foundation for implementing various features and functionalities in web applications.
Why Send Custom HTTP Headers with cURL?
Custom HTTP headers serve as powerful tools for developers. It allows them to simulate different scenarios and interact easily with APIs. Whether you’re testing a new feature, debugging an issue, or integrating with a third-party service, custom headers enable you to tailor your requests to specific requirements.
For instance, imagine you’re working on an application that interacts with a weather API. By sending custom headers specifying location coordinates, units of measurement, or language preferences, you can fine-tune the API responses to match your application’s needs. This level of customization empowers developers to create more robust and adaptable solutions.
Additionally, custom headers are invaluable for testing edge cases and error handling. By manipulating headers, developers can simulate various network conditions, server responses, and authentication scenarios, ensuring that their applications are resilient and capable of handling real-world challenges.
Advantages of Customizing Requests with cURL
cURL provides developers with a straightforward yet powerful interface for customizing HTTP requests, making it a preferred choice for interacting with APIs and accessing restricted resources. By leveraging cURL’s flexibility, developers can easily include custom headers to authenticate themselves, authorize access, and unlock additional functionalities.
Authentication is a common use case for custom headers, especially when working with APIs requiring API keys, OAuth tokens, or other credentials. By adding authentication headers to their cURL requests, developers can securely access protected resources and perform authorized actions on behalf of authenticated users.
Moreover, custom headers enable developers to bypass access restrictions and access resources that would otherwise be unavailable. For example, some APIs may impose rate limits or require specific permissions for certain endpoints. By including appropriate headers, developers can circumvent these restrictions and access the desired resources without compromising security or violating API terms of service.
Flexibility and Control Offered by Sending Custom Headers with cURL
One key advantage of using cURL to send custom headers is the unparalleled flexibility and control it offers developers. With cURL, developers have full control over every aspect of their HTTP requests, from the request method and URL to the headers and payload.
This flexibility allows developers to experiment with different configurations, fine-tune their requests, and optimize performance based on specific requirements. Whether it’s adjusting caching directives, specifying content types, or controlling compression settings, cURL empowers developers to tailor their requests to achieve optimal results.
Furthermore, cURL’s command-line interface makes automating repetitive tasks easy, integrating with scripts and workflows and streamlining development processes. By encapsulating HTTP requests in cURL commands, developers can quickly prototype ideas, debug issues, and deploy solutions with minimal effort.
In summary, sending custom HTTP headers with cURL offers developers unparalleled flexibility, control, and convenience. This makes it a vital tool for modern web development.Â
Basic Steps for Sending HTTP Headers with cURL
Sending HTTP headers with cURL is a fundamental skill in web development. It allows you to customize your requests and interact with web servers efficiently. Let’s walk through the basic steps for sending HTTP headers using cURL, enabling you to craft requests tailored to your needs.Â
Specify the URL for the Request
The first step in sending HTTP headers with cURL is to specify the URL for the request. This URL indicates the destination server or resource you want to interact with. You can specify the URL directly in the cURL command followed by the desired HTTP method (e.g., GET, POST, PUT, DELETE).
Example:
curl https://api.example.com/resource
In this example, we’re sending a GET request to https://api.example.com/resource.
Add Custom Headers Using the -H or –header Option
Once you’ve specified the URL, you can add custom headers to the request using the -H or –header option, followed by the header name and value. This option lets you include one or multiple headers in your cURL command.
Example:
curl -H “Content-Type: application/json” -H “Authorization: Bearer <token>” https://api.example.com/resource
Here, we include two custom headers: ‘Content-Type’ set to ‘application/JSON’ and ‘Authorization’ set to a bearer token.
Execute the cURL Send Header Command to Send the Request
After specifying the URL and adding custom headers, you can execute the cURL command to send the request. To do so, simply type the cURL command in your terminal or command prompt and press Enter.
Example:
curl -H “Authorization: Bearer <token>” https://api.example.com/resource
This command sends a GET request to https://api.example.com/resource with an Authorization header containing a bearer token.
In summary, executing the cURL command triggers the HTTP request, and cURL displays the response received from the server in your terminal or command prompt. You’ll see the response body and relevant HTTP headers, allowing you to inspect the server’s response and handle it accordingly in your application.
These basic steps provide a straightforward approach to sending HTTP headers with cURL, allowing developers to customize their requests and interact with web servers efficiently. Whether you’re testing APIs, debugging network issues, or integrating services, mastering these steps will empower you to tailor your interactions with precision and control.
Advanced Techniques for Sending HTTP Headers
While learning web development, mastering advanced techniques for sending HTTP headers can greatly improve your capabilities. While basic header customization is essential, using advanced techniques allows you to customize your requests, optimize performance, and tackle complex scenarios precisely. Here, we’ll explore advanced techniques for sending HTTP headers using cURL. This includes:Â
Sending Multiple Headers in a Single Request
Sending multiple headers in a single cURL request allows you to include additional information without cluttering the command line. You can achieve this by specifying the -H or –header option multiple times with a different header.
Example:
curl -H “Content-Type: application/json” -H “Authorization: Bearer <token>” https://api.example.com/resource
In this example, we’re sending two headers (Content-Type and Authorization) in a single request to https://api.example.com/resource.
Reading Headers from a File for Improved Organization and Management
Reading headers from a file can improve organization and management when dealing with many headers or complex configurations. Instead of specifying headers directly in the command, you can store them in a text file and reference them using the -H option with @ followed by the file path.
Example:
curl -H @headers.txt https://api.example.com/resource
The ‘headers.txt’ file contains each header on a separate line in the format ‘HeaderName: Value’. This approach simplifies command-line inputs and facilitates collaboration by allowing you to manage headers separately from the cURL command.
Ignoring SSL Certificate Verification for Specific Cases
In some cases, such as testing or development environments, you may need to ignore SSL certificate verification to proceed with the request. While it’s generally not recommended for production use due to security implications, cURL provides the -k or –insecure option to ignore SSL certificate verification.
Example:
curl -k https://insecure.example.com
Therefore, using this option, cURL bypasses SSL certificate validation and proceeds with the request. This further allows you to interact with servers with self-signed or expired certificates. However, it would be best to exercise caution when using this option in production environments, as it may expose your connections to potential security risks.
These advanced techniques offer additional flexibility and control when sending HTTP headers with cURL, empowering developers to customize their requests efficiently and easily handle diverse scenarios. Whether you’re sending multiple headers, managing headers from a file, or bypassing SSL certificate verification, mastering these techniques enhances your ability to interact with web servers effectively.
Integrating NetNut Proxy Service with cURL Send header
When discussing web development, the ability to send HTTP requests with ease and precision is important. Whether undergoing the data extraction API process, testing endpoints, or scraping web content, having the right tools at your disposal can make all the difference.Â
Integrating the NetNut proxy service is necessary to improve the cURL send header process. NetNut is a powerful residential proxy service provider that makes sending HTTP headers using cURL easy and fast.Â
Combining NetNut proxy tools can enhance your ability to craft custom requests, access restricted resources, and optimize your web development workflow.
Why Integrate NetNut with cURL?
Integrating NetNut with cURL opens up a lot of opportunities for developers. These benefits include:Â
- Anonymity: NetNut’s residential proxies ensure your requests appear as though they’re coming from real residential devices. Therefore, they enhance anonymity and reduce the risk of detection or proxy block.
- Geolocation: By routing requests through NetNut’s global network of residential IPs and mobile proxies, developers can access geographically restricted content and simulate user behavior from different locations.
- Reliability: NetNut’s high-performance infrastructure guarantees fast and reliable proxy connections, minimizing downtime and ensuring a smooth development experience.
- Scalability: NetNut’s scalable proxy infrastructure allows developers to handle large volumes of web traffic and scale their applications without worrying about proxy limitations.
Step-by-Step Guide to Integrating NetNut with cURL
Here’s how you can integrate NetNut with cURL to send HTTP proxy headers with ease:
Sign Up for NetNut
Start by signing up for NetNut and obtaining your API credentials. You’ll need your username and password to authenticate your requests using NetNut’s API.
Configure cURL with NetNut Proxy
Use cURL’s—-proxy option to specify the NetNut proxy endpoint and port. The proxy URL should also include your NetNut username and password.
Example:
curl –proxy http://<USERNAME>:<PASSWORD>@<PROXY_ENDPOINT>:<PORT> https://api.example.com/resource
Add Custom Headers
Like regular cURL requests, you can add custom headers using the -H or –header option. Include any additional headers required for your request, such as authentication tokens or content types.
Example:
curl -H “Authorization: Bearer <token>” –proxy http://<USERNAME>:<PASSWORD>@<PROXY_ENDPOINT>:<PORT> https://api.example.com/resourceÂ
Execute the Request
Once you’ve configured cURL with NetNut proxy and added your custom headers, execute the cURL command to send the HTTP request. NetNut will route your request through its residential proxies, providing you with anonymity and bypassing any restrictions.
Whether you’re testing APIs, scraping web content, or automating tasks, NetNut’s integration with cURL provides a powerful solution for enhancing your web development capabilities. So why wait? Optimize NetNut and cURL integration today!Â
Conclusion
On a final note, in the process of web development, mastering cURL and understanding how to send HTTP headers easily is a powerful tool that opens doors to endless activities. By customizing HTTP requests with headers, developers gain total control over their interactions with web servers.Â
Whether it is authentication, accessing restricted resources, or fine-tuning request parameters, cURL empowers developers to tailor their requests to specific requirements precisely and easily. The importance and benefits of sending HTTP headers with cURL cannot be overstated. Custom headers enable developers to simulate different scenarios, interact smoothly with APIs, and optimize performance.Â
By integrating techniques explained in this guide, you can improve your skills with cURL. In addition, they tackle complex challenges and deliver innovative solutions that push the boundaries of what’s possible on the web.
So, embrace the power of the cURL send header, and let it be your trusted ally on the path to building exceptional web experiences. With cURL by your side, the possibilities are truly limitless.
Frequently Asked Questions and AnswersÂ
Is it possible to send headers with cURL in a POST request?
Absolutely. Whether it’s a GET, POST, PUT, or any other HTTP method, you can include custom headers with cURL using the -H option. You can also specify the request payload using the—d or—-data option for a POST request.
Can I use cURL to test REST APIs?
Yes, cURL is commonly used for testing REST APIs due to its simplicity and versatility in crafting HTTP requests with custom headers. You can send requests (GET, POST, PUT, DELETE) and include custom headers to test different API endpoints and functionalities.
Are there any limitations to the length or number of headers I can send with cURL?
cURL does not impose strict limitations on the length or number of headers you can send. However, practical limitations may apply based on system resources, network constraints, and server configurations. It’s always a good practice to keep the headers concise and relevant to avoid potential issues.
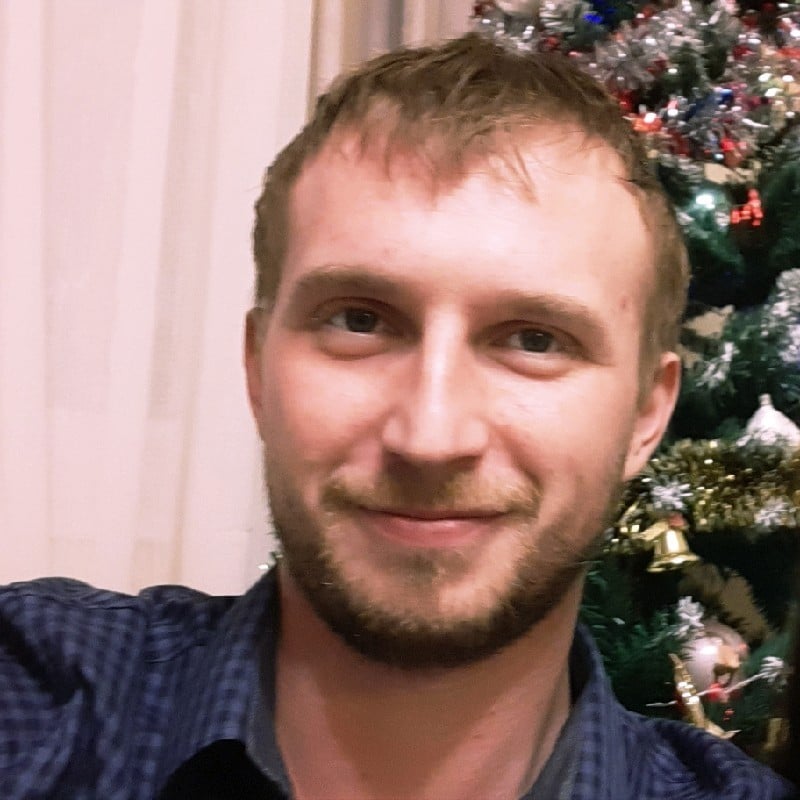